1. What is type conversion?
Type conversion is the conversion of one type to another type. Conversion can be divided into implicit and explicit conversion.
Implicit conversion is when there are different types on both sides of the operator. The compiler will try to implicitly convert the types without losing data.
For example: uint8 -> uint16, uint256 can be converted
uint16,uint256 -> uint8 will not be converted automatically because data may be lost
Explicit conversion is forced conversion through code, such as the conversion of uint16 to uint8 above. If you know that there is no problem with the variable conversion, you can force the conversion yourself.
For example, uint256 num = 100; uint8(num); can be forced to convert to uint type
Be careful with forced conversions. If an incorrect conversion is performed, an error will be reported. If a variable is converted to a smaller data type, the excess part will be truncated.
2. delete reset variables
The delete operator is used to reset variables. Note that delete is invalid for mapping types (elements in mapping can be deleted). Delete does not affect the value of the copied variable.
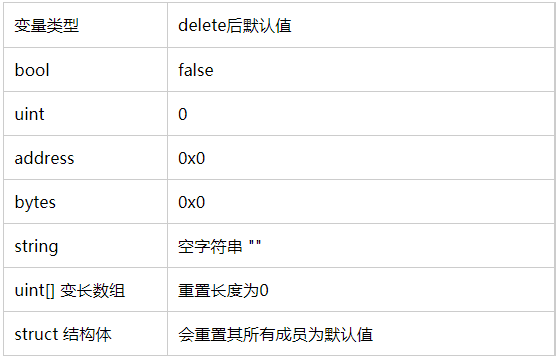
3. Practice writing code
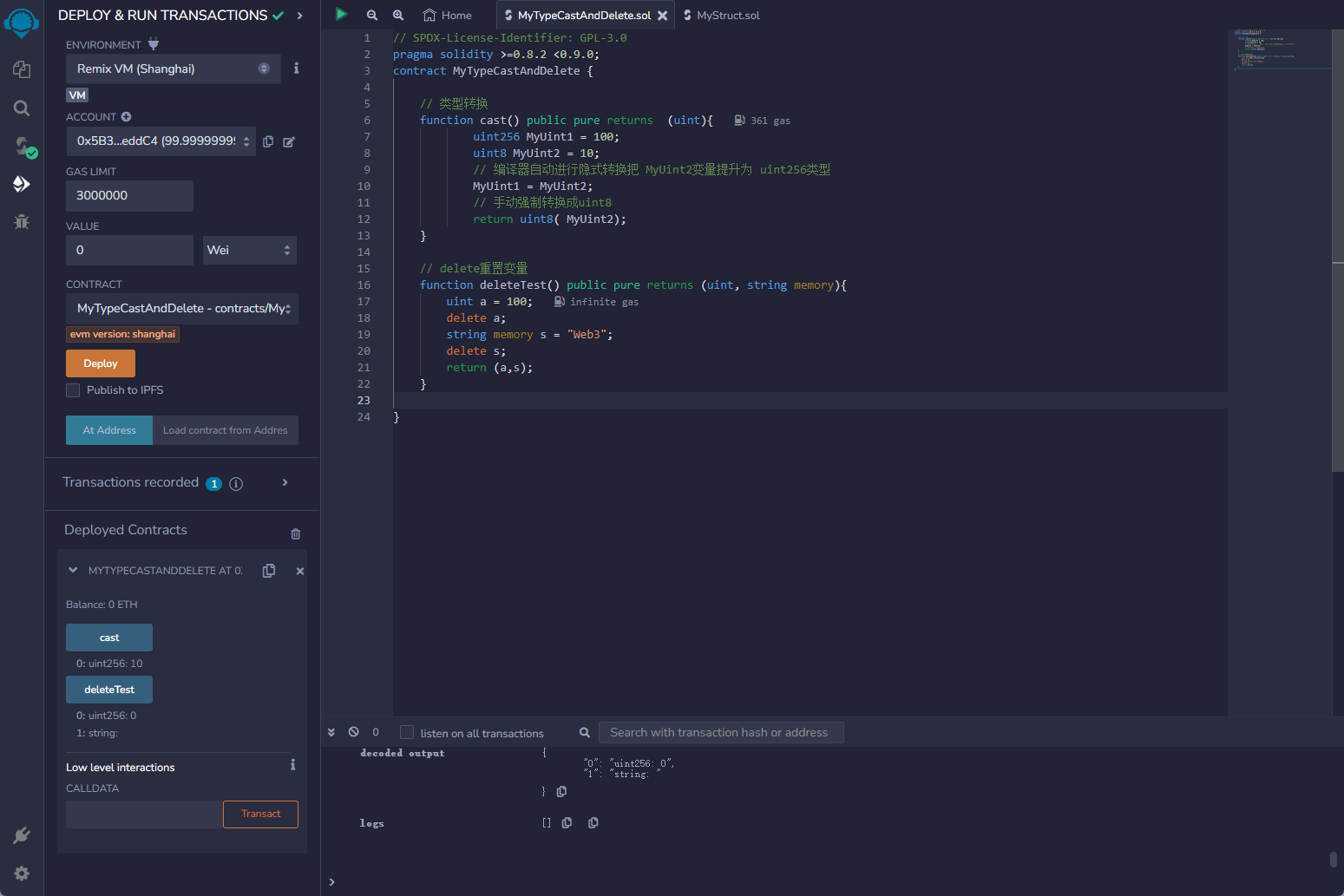