1. Keyword struct custom type
struct Player{
string name;
uint coins;
}
2. Declaration and initialization of structures
1. Only declare variables, do not initialize them
Player public player;
2. Initialize in member order. If the structure has mapping, it needs to be skipped.
Player public player = Player(1,"Tom",1);
3. Initialize by specifying the member name, so there is no need to specify the order of the members. When there are many structure variables, this is recommended
Player public player = Player({id:1,level:1});
3. Access and assignment of structures
The structure uses dot notation to access members, for example: xxx.name, and the same is true for assignment: xxx.name="Tom"
players[msg.sender].name = name;
4. Restrictions on structures
Currently, structures can only be used within contracts or in inherited contracts. If you want to use structures in parameters and return values, the function must be declared internal. This problem does not exist after version 0.8.
5. Let’s practice writing code
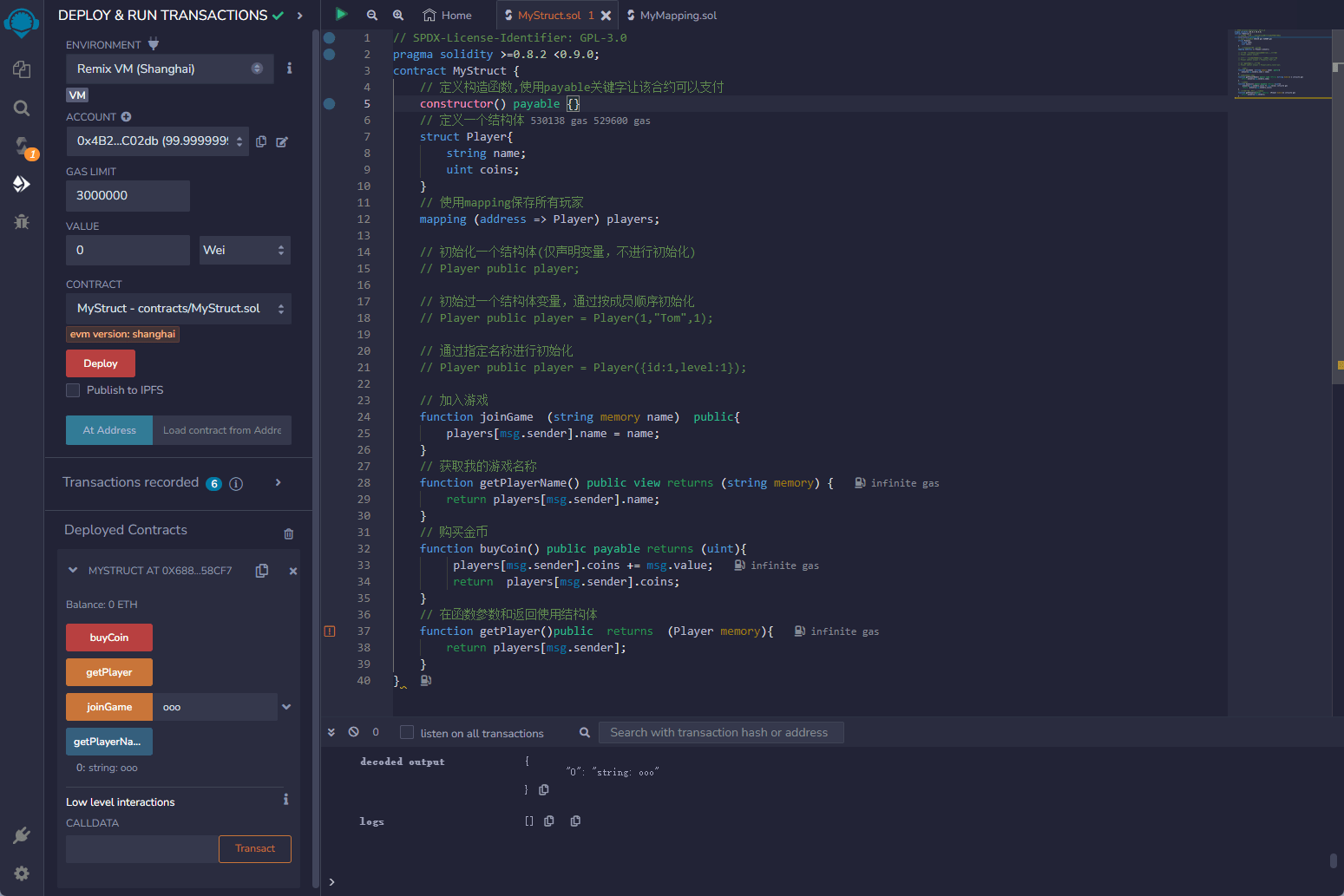